I keep remembering that good ideas start from doing something fun, instead of doing something easy. About a month and a half ago I was reading the Google Doc that I have with some friends, listing ideas about “Changing the World”. It’s all the things that should/could be better in the world and “I wish….” it would be some way.
In the list of a hundred or so I’ve seen one entry that was wishing for a way to see changes to our shared documents in an easier way than going back to the Docs home page and seeing if something has happened. Been thinking that that would be something I myself would totally use. Couldn’t find anything like that yet, but I had sometime in my hand to experiment, so WatchDoc was born….
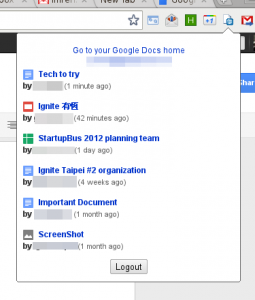
Getting it done
I haven’t written a Chrome Extension before, but seemed like such a suitable fit, and been looking for a project that I can try myself with. They are practically open source (since you can look into any one of them regardless of where you installed them from) and only using standard web tech, just like making a website (HTML + CSS + JavaScript). Indeed, all Chrome extension are some web-pages displayed in a special way, some javascript running in the background and/or javascript modifying the page you are on. Very neat, I would say…
Since I didn’t know where to start, I tried to find an extension that I can take apart and learn from. Fortunately, there was one, straight from Google, called YouTube Feed. So the first part of the project was slowly gutting that one out and replacing parts until I get something working that I wanted. It is easier to say than do, because the feed reader has somewhat different requirement than the code I had in mind, but at least close enough that most of the internal structure I could keep.
Some notes about the journey:
- Authentication: oAuth can be pretty troublesome, but in the end it was easier this time than I expected from past experience. Maybe because YouTube Feed was written reasonably well already
- Icons: Wanted to take the icons for the different document types from Google Docs itself. Took some time, but using the developer tools (inspect element) in Chrome I could finally find the links and download them.
- More icons: Wanted to use the icons as a CSS image sprite just like the original extension, but couldn’t find some good program to combine PNG files into a single image. In the end wrote a quick Python script to do just that.
- Extension icon: For the extension icon I used Google Docs’ own little icon (I think it’s kinda fair to use, especially now that they have changed it, it’s v9 of their icon theme compared to v7 at the time of my programming) and also on a free icon site I had found a matching hi-def icon. I just wish I could find it again for proper attribution, that still nags me.
- Buggy notification: Some parts of the extension writing was pretty annoying. For example I wanted to get desktop notification work just the way it does in Gmail. I don’t know how do they do the auto-hiding, but I’m pretty sure not from the standard simple desktop notification type, because that I simply make to hide itself after a certain time. Instead I had to make it the more difficult way of using a HTML page to define content and then internal JavaScript to close it. Took a while to realize that I have to pass my parameters (the data that I wanted to display) as query parameters, but now it works pretty well.
- Buggy libraries: this is always fun to have because have to find to work around it or fix it. This time it was a jQuery URL Parsing library that crapped out on corner cases that the developer didn’t thought of but I fortunately run into. Took a while, but fixed it up, let’s see if upstream will incorporate it.
- Optimization: it’s good to have things working first and then have them work well. For example originally I re-read the user’s whole feed, now it can read the part of the update feed that can contain relevant information, so I can have fewer requests to the server, and the updates come in several seconds quicker.
Release
After some work I thought it’s better to release first and ask questions later. So I registered on the Chrome Web Store (registration is $5 on time fee to get rid of spammers). Not much hustle, in about 20 minutes everything is done and now you can find WatchDoc in the Chrome Web Store. You can also find the source on GitHub.
The Chrome Web Store seems to have some strange policies, though, eg. didn’t let me update if I didn’t have the right shapes of preview pictures, and sometimes they only told me halfway into the submission of the new release, and I had to scramble to make some test documents and nice screenshots of the right aspect ratio so I can finish the submission. Nevertheless it is pretty easy to handle and useful too.
One beef I have is that I don’t have any way to reply to reviews. Much more people post negative stuff than positive and once I fixed something there’s no way to get in touch with the reviewers to ask for re-evaluation. This makes a very one-sided experience.
It is good – though addictive – to watch the number of +1s grow, as well as installs and users. The site must have some way to check people’s installations because the total number of users did decrease after the initial increase (the high water mark somewhat above 1500, now a bit below 1400). I guess I would need to improve the quality a bit more, and maybe there are not that many people sharing Google Docs with others than I have thought. :) Anyway, I think this is much more than how many people used any other software/site I made, so no ground to complain.
Post-release
The release was picked up surprisingly quickly by other websites, I think there are some automatic ones monitoring the latest submissions to the Web Store, and others take the news from them.
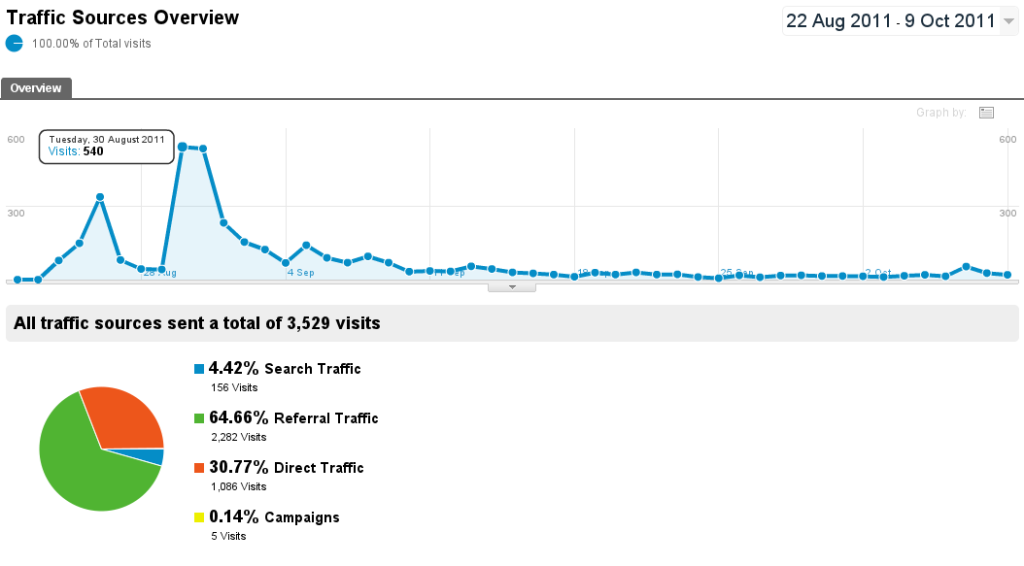
Here’s my Google Analytics since the release. The big spikes in the beginning go up to about 500 visit / day (tiny, but much more I have ever had), and are mostly due to Lifehacker. After that I have basically just a trickle of visitors (about 20 / day), with a little spike recently that is direct traffic, I wonder where from….
Some sites that reviewed WatchDoc (the ones with the highest referral counts)
- Addictive Tips, these guys seem to be monitoring the Web Store submissions and react pretty quickly, basically within hours of submission without me advertising it anywhere
- How-To Geek, they copy the previous one
- Korben, doing well in French
- Techstatico, doing well in Spanish
- Betashuffle
- Lifehacker, later on Lifehacker.ru and Lifehacker.jp, and some other regional sites with smaller number of visitors
- Stadt-Bremerhaven, this one is surprising me, the website of the city of Bremerhaven, must be very nerdy people there, should go and visit
- cnet/How-to, in a compilation of useful apps
- thenextweb, in another compilation of useful apps
- TechZilla Daily, got my own video review :) that’s neat!
I got up a support site on GetSatisfaction as well to be able to have a conversation with those who are persistent enough and submit some feedback regarding bugs. Used it a couple of times, and it’s quite practical. I finally learn how to do product support, which doesn’t mean it became easier. Still surprisingly maddening to debug a problem until it hits me as well by chance so I can check it locally. Got to figure out some superior remote debug system. At this point it seems to work well enough for about 1400 people, but the fact that I have lost about 200 shows that I still have a lot of problems to fix.
Into the future
It was a fun project to work on and it does most of the things I wanted to, but it’s dead ugly. I either find a designer and fix it, or just leave it like this because it doesn’t matter much…
I might work on it a bit more, especially bugfixes, though it would be better to have some ideas what is missing. Everything I can think of would complicate the user experience and that’s not my plan: document preview, ignore updates of certain documents, multiple-logins,…. What else?
Guess it is more likely that soon I will start to work on something else, let me know if you have something fun you wish to see :)