I’m a few days behind schedule since Lua was June’s language, but here it comes, some summary of my experience, after setting things up in the first part.
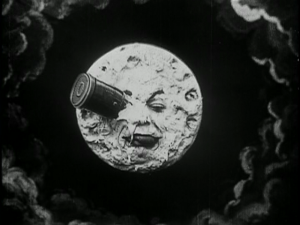
Overview
I really liked this language, and I’m sure I’ll keep learning it. Very useful and lots of interesting concepts. I haven’t seen an embedded language before, and some parts
Most of what I picked up was from Beginning Lua Programming and Programming in Lua. Lua Missions was another very useful experience: a “fill in the blank” type of source code collection, which drilled my understanding of the Lua concepts. Some sections felt a little short, so maybe later I’ll think whether there are any more questions I can come up with. Very well worth the time!
There are two things that stand out: tables and local environments.
Tables
If I have to refer to my knowledge of Python, then Lua tables are like Python lists and dicts rolled into one and then some. Here’s one such table:
x = {1, 2, 3, y = "something", ["or else"]="what"}
The first three elements are giving an array (and one can loop over them), then the last two are key-value pairs. One tricky thing is that the “y” there is actually a string (the same as [“y”]), just made it more readable with syntactic sugar. Since variables (references to variables) can be keys as well, this can get confusing if one has a variable called “y”… There are quite a few things about tables that are confusing, but actually pretty consistent so my fuses are not blowing that much as they were in the beginning (for example: how the length is looked up, how to iterate through the different values) .
They are very complex animals so some things are quite complex to do, which in turn enables quite complex things to be done. Pretty much any kind of data structure can be built upon them. So all in all, I’m enjoying them with all their quirks. Also, “weak tables” are an interesting concept (where certain table entries can garbage collected).
Scopes
In Python, the name spaces and environments seem to be on the sidelines (at least what I’ve seen so far): people need it and have to us it, but nothing more than that. In Lua this is taken to a whole new level: scopes can be essential parts of the way algorithms are implemented and neat tricks can be played.
I tried to come up with some good example and failed, here’s a “duh” one:
function newCounter ()
local i = 0
return function () -- anonymous function
i = i + 1
return i
end
end
c1 = newCounter()
print(c1()) -- 1
print(c1()) -- 2
This is a bit like Python’s “yield” type of generator, without anything special.
Also, in Python there are only two kinds of variables (I think): local and global: either just for you or for everyone. Here they have one more: upvalues, where the variable is local to a parent, so children environment can access them but the parent’s parents cannot. They are strange beasts as well, e.g. the code itself cannot really know if a variable is upvalue or global, only that it can access that.
And of course these scopes help embedding to not just be possible but straightforward and powerful. Local environments can be restricted, some things selectively enabled, and all this transparently, so the script running might not even know that it’s being in the Matrix.
The Lua Experience
Good
- Fast, both start up and running
- Language itself is quite small, possible to wrap one’s head around quite easily
- Written in ANSI C, so basically on every architecture where one can run C, one can have Lua as well
- Quite a few program has Lua interpreter or interface built in, see the list of these at Wikipedia
- Tables are great and once one mastered them, are really Swiss Army Knives
- Built in interpreter (though could use a little love like iPython)
- The “…” syntax for variable number of arguments
- Astrological naming convention – modules are rocks, the exercises I linked previously are missions, web framework is Kepler…
Bad
- There seem to be a lot of reserved names (e.g. in metatables) that would be pretty much impossible to figure out with a book (and/or an iPython-like helpful environment), though this is a problem probably only when starting out with Lua
Ugly
- While some complex things can be solved, some simple things can require complex coding (e.g. in case of the tables when using “__index” and “__newindex“) and there are things that are not even possible. Or I just misunderstood some sections of the book.
- Using a lot of syntactic sugar (especially in the object oriented section), that I feel need some more practice to pick up, as things can be done in multiple ways
- The tables’ syntactic sugar covered way of handling string keys, convenient when things go well, mighty confusing at even the slightest problem
- Too few projects using Lua, too few sources of information (this is not the language’s fault, though)
Project
Originally I wanted to do some useful programming in every language I learn during the Language of the Month series. I failed with Scala (lazy), and now failed with Lua too (underestimating the difficulty). I’m using VLC a lot and it has Lua scripting enabled, so I wanted to do a plugin that can be useful for me later as well. This didn’t work out very well, mainly because it feels the VLC Lua interface is not very well documented, one mostly have to find some examples that exist already and use them. I ended up wasting a lot of time and still don’t quite understand how to do certain things I would like for my code, and don’t even know if it’s possible to do or not. Will come back to it later, until that here are a few links that helped me to get started:
- VLC Lua FAQ – detailed list of available API functions, but almost no context and examples
- Lua extensions in the VLC git repo – one can pick up something by checking out those examples
- Example: Streaming Radio Player Extension – someone who have gone further down this road than me and has a working extension. Very educational, especially to see how concise and straightforward it can be, because the VLC Lua API seems to be thought out well.
Again, hopefully pick this up later (I mean it).